The Mysterious Behaviour of the Filter Box in DbViewSharp
How often are you baffled by the way a particular feature in a program works? Sometimes it will do this, but it is equally likely to do something else in what seems to be the same situation. Of course the situations are not the same in both cases from the point of view of the program, or more accurately the programmer who designed and developed the feature, but that does not help you if you do not have an in-depth knowledge of the workings of the program. As a consumer of software written by others I should know better. I'm guessing that DbViewSharp is packed full of such features; blindingly obvious and really clever to me as designer, developer and chief user; infuriatingly fickle to anyone else just trying to to get a job done. This post will take a detailed look at one of these features, the Filter Box, and describe it in depth so that you can come to regard it the same way that I do, as an incredibly versatile timesaver.
First of all what is the Filter Box? Well its the drop down combo box that sits above the main grid.
You first notice it when you connect, because it flashes red until the table list is displayed. This is just an overload of the control to act as visual feedback when a slow query is running. Once the grid is displayed then entering text into the box and hitting Enter or pressing "Go" will change the grid display by using what you just entered as a row filter.
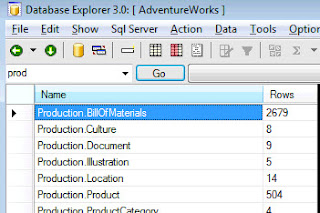
When the table names are displayed the filter will match on the table names. However I am trying to apply more of a browser style search than a strict SQL query to the matching. By this I mean that instead of having to enter exactly what you want to search on, taking care of case and punctuation (punctuation in this sense refers to underscores in table names), you just need to enter a substring that your target table name or names contain and which will limit the grid to rows with just those tables you are interested in. Like Google you may see items in the grid that you are not interested in, but just like Google with a tiny bit of practise and basic knowledge of your schema you soon discover the minimum effort required to bring your tables into view from even a large list. Google does not require you to enter more than a few keywords. DbViewSharp does not (normally) require more than a few characters. Google matches the keywords anywhere in the page. DbViewSharp matches the characters anywhere in the table name. Unlike Google however DbViewSharp only searches correctly for a single substring. If you try entering two separate substrings nothing will match as the two are treated as a single substring with a space in it.

Things get more complicated when you are displaying data from a table. Here is where the fun starts. It's fun because you don't appear to get a chance to explain to DbViewSharp what columns you want the filter to act on, yet it goes ahead and filters the rows anyway. Sometimes, bafflingly, it doesn't filter any rows. So lets try to change this from confusing to genius.
Unlike the tables list there could be many columns of table data in the grid so which of them are used in the filter search? It will help you to understand what is going on if you right-click in the top left corner of the grid following a filter search. This causes a context menu to appear from which you can select "Show SQL". This in turm does just as you'd expect. It reveals exactly how the filter text is put to use to search the grid data. Here's an example from one of my test databases.
select top 500 [id],[name],[class],[typeof],[fb_id],[description],[STATUS]
from Animals
where ([name] like '%bat%' or [class] like '%bat%')
Note that two columns were used in the search apparently at random. However it is not random. The parameters for the grid display were generated automatically when the table was double-clicked. The routine that decides on the filter columns examines the first three columns in the table and assigns them to either a text filter list or a number filter list depending on their type. The reasoning is that normally the most important and searchable columns are added to the table first. Look at my example. The first three fields seem pretty important, particularly id and name. The id is very likely to be a numeric key field and so is excluded from an alphabetic search.
When I entered "bat" in the text box I was interested to find rows of bats, which I get and without writing a single line of SQL. I'll repeat that. I found the bats in the table without: 1) remembering the SQL syntax; 2) typing in any keywords; or 3) writing a fiddly where clause. I simply typed "bat" into the filter box and pressing "Go". It's the same when filtering on numeric data. If you enter a number in the filter box it assumes you are looking for a key or keys with the value entered. So if you know the id of a record just enter the number in the filter box and hit Enter. If the id is good you should see your record.
select top 500 [id],[name],[class],[typeof],[fb_id],[description],[STATUS]
from Animals
where ([id] = 7002)
Another common search requirement using keys is to search a line-item table for all rows related to a master record (think Customer orders or Invoice line items). As long as the master record Id is in the first three fields of the line-item table you will get this type of look-up for free as well.
The following situation has been a common scenario in my professional life. Given the ID of a record that has a problem find the cause and fix it. Without DbViewSharp this is the start of an awful lot of ad-hoc SQL writing. With DbViewSharp I start by checking the problem record in DbViewSharp like so:
- Connect to the database to obtain the table display.
- Locate the table name using the filter box.
- Double-click to view the data.
- Enter the record id in the filter box.
- Double click on the record line to view the data in profile (ie. one row per column).
- Confirm that the error is in the data, then scan for other clues and continue to hunt through data and stored procedure code using DbViewSharp until the job is done.
The big gain, I think, is that once you are familiar with how to use DbViewSharp and the way the filter box works then you do not not need to context-switch from thinking about your trail to the cause of the problem and how to write the SQL you need to get the information you need to continue on the trail.
What if the automatic filter column selection lets you down? Suppose the master record Id is not selected as a number filter. You can modify the filters using the Data, Change view option or pressing the toolbar button that looks like a window, located to the left of the funnel icon. Select fields from the DataView Fields list and use the relevant [>] and [<] buttons or drag and drop to add or remove them from the Text filter and Number search lists.
Changes you make to the automatic DataView will remain in place until you quit DbViewSharp. If you find yourself repeatedly changing the filter fields consider saving them as a new DataView.
Now you have a clearer picture of how the filter box works on searching the data I need to describe how to manage the filter box itself to achieve maximum fluency.
- If the focus is on the grid then when you type anything the focus switches to the filter box and the text is displayed there for searching. The grid gains focus after it is displayed.
- Use the escape key to clear the filter box and redisplay the grid unfiltered.
- Previous search text is added to the drop down. The list is maintained in last-used order. This is massively useful when tracing records with the same Id over multiple database.
There is subtle logic used to determine whether to use the filter text or clear it. If you have just applied the filter search to one set of objects and are switching to another then you probably do not want the filter to be applied to the new object list (eg. switching from filtered tables to views). If you have just entered a new filter and are switching then you probably do want to apply the filter otherwise why did you bother to enter anything?. However there is a common scenario where this logic is wrong. This is the case where you enter filter text and search only to realise that you just searched the wrong set of objects. For example in your mind you are searching the list of views, but the grid was actually displaying tables. When you switch to views your search disappears and the full list appears. You can recover the search text from the list connected to the filter box, but I found this happening so often I programmed a short-cut for it. The clear search toolbar icon (looks like a thin white rectangle) behaviour is now to clear the text box and restore the full list if it contains text, but, importantly, to restore the previous search text and apply it to the current grid if it does not contain text. That is the last piece of the jigsaw that enables this smart filtering to work so well.
Final tips
Occasionally you may be frustrated that you cannot search for numeric data in text columns because numbers entered are matched to numeric columns. The workaround for this is to explicitly include % in the search text, but then it uses proper SQL rules; so %10% (anywhere), 10% (starts with) or %10 (ends with) will match correspondingly: "Sport-100 Helmet", "10-speed aluminum derailleur" or "Hex Nut 10".
You can include a % in non-numeric searchs and the same rules apply. The most common case is to search for items starting with the text. This may be useful if your objects follow a naming convention that uses prefixes or schemas. In the MS AdventureWorks sample database for example, you can filter just the HumanResources tables with "H%".
Conclusion
The filter box originated from my profound irritation with the way that SQL Server Management Studio requires you to type without pauses and without mistakes to navigate the table lists. It was then extended and refined over years of (personal) use to become the means by which I can satisfy at least 90% of my data-searching requirements in DbViewSharp. I hope this post has given you the encouragement to experiment and get to understand it a little better. Don't forget to look at the SQL.
DbViewSharp is an open source desktop application that connects to SQL Server databases and allows you to search for just about anything important. Find
DbViewSharp on Codeplex.